Animated texture and billboard
Before proceeding to "Steps to implement" described in this section, make sure to construct your effect with the appropriate ".fbx" model in the Filter Editor. Refer to the section Animated 3d mask.
The Filter Editor allows for the following operations being implemented before the manual effect customization:
- Enable backfaces for billboard materials.
- Set "blend" value to "alpha" for billboard materials if they have alpha channel data.
#
Sprite billboard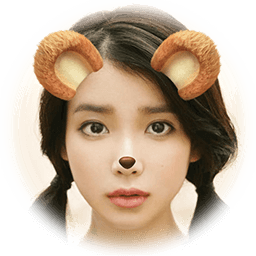
Effect example: bear
#
Steps to implementPut 2 shader files into the effect folder:
- billboard.vert
- tex.frag
In cfg.toml file change the shader values for the materials which are supposed to be a billboard with the sprite texture:
- vs = "billboard.vert"
- fs = "tex.frag"
In cfg.toml file in the material's "samplers" section remove all the statements except for "tex_diffuse" which is the sprite texture.
#
Animated texture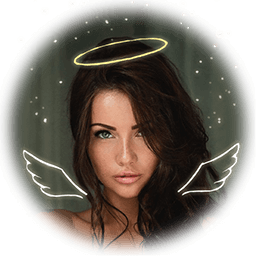
Effect example: angel
Only ".mp4" files are supported as an animated texture. You can use 2 alternative fragment shaders for the animated textures:
- video.frag shader for video files without the alpha channel data. Used with one of these "blend" option values: screen, add, multiply.
- video_a.frag shader for video files with the alpha channel data. The width of such a video file must be twice larger than the original video file. The left part of the video is the texture and the right part is the alpha channel data.
#
Video textures conversionYou can use FFMPEG as a converter tool: https://www.ffmpeg.org
Recommended usage:
Options:
Option | Description |
---|---|
-i file | input file |
-crf value | Set the value for the constant quality mode (from 0 to 63) (default 0). For optimal results, we recommend using the values of about 21-26. |
Example:
You can call "ffmpeg -h" to get a more detailed description of the tool. We recommend using the uncompressed or lossless input files in order to avoid unwanted artifacts in the final video.
#
Steps to implementnote
The animated billboard textures require at least one keyframe on a video.
Put 2 shader files into the effect folder:
- billboard.vert
- video_a.frag
In "cfg.toml" file change the shader values for the materials which are supposed to be a billboard with an animated texture:
- vs = "billboard.vert"
- fs = "video_a.frag"
In cfg.toml file remove all statements in the "samplers" section.
Add "media" section into config.json inside "frx" section. Use ".mp4" file.
Uncomment the line below in config.js to enable the animation playback: